Tinkering with Window Gaps in dwm
Introduction
Hello fellow hackers! Following on from my previous blog post , Today, I had a bit of fun customising the gaps between windows and the edges of the monitor in the Dynamic Window Manager. I'll talk about the process of modifying the previous resizeclient
function to achieve uniform gaps and larger edge gaps for a more visually appealing layout.
What is the resizeclient
Function?!
The resizeclient
function is responsible for resizing and repositioning windows. It takes the following parameters:
parameter | Usage |
---|---|
Client *c |
A pointer to the client (window) to be resized. |
int x, y |
The new x and y coordinates for the top-left corner of the window. |
int w, h |
The new width and height for the window. |
This is how the code used to work:
void resizeclient(Client *c, int x, int y, int w, int h) {
XWindowChanges wc;
unsigned int n;
unsigned int gapoffset;
unsigned int gapincr;
Client *nbc;
wc.border_width = c->bw;
/* Get number of clients for the client's monitor */
for (n = 0, nbc = nexttiled(c->mon->clients); nbc; nbc = nexttiled(nbc->next), n++);
/* Do nothing if layout is floating */
if (c->isfloating || c->mon->lt[c->mon->sellt]->arrange == NULL) {
gapincr = gapoffset = 0;
} else {
/* Remove border and gap if layout is monocle or only one client */
if (c->mon->lt[c->mon->sellt]->arrange == monocle || n == 1) {
gapoffset = 0;
gapincr = -2 * borderpx;
wc.border_width = 0;
} else {
gapoffset = gappx;
gapincr = 2 * gappx;
}
}
c->oldx = c->x; c->x = wc.x = x + gapoffset;
c->oldy = c->y; c->y = wc.y = y + gapoffset;
c->oldw = c->w; c->w = wc.width = w - gapincr;
c->oldh = c->h; c->h = wc.height = h - gapincr;
XConfigureWindow(dpy, c->win, CWX|CWY|CWWidth|CWHeight|CWBorderWidth, &wc);
configure(c);
XSync(dpy, False);
}
Making Gaps Look Just That Bit Cooler
To make things a bit more interesting, I decided to introduce an additional variable edgegap to make the gaps at the edges of the monitor slightly larger than the gaps between windows. We also adjusted the calculations to ensure uniform gaps on all sides.
Now, we've changed it to this:
void resizeclient(Client *c, int x, int y, int w, int h) {
XWindowChanges wc;
unsigned int n;
unsigned int gapoffset;
unsigned int gapincr;
/*
* For some reason, I feel like the window gaps are larger than edges
* so let's introduce edgegap's so I can modify them to my hearts content.
*/
Client *nbc;
wc.border_width = c->bw;
/* Get number of clients for the client's monitor */
for (n = 0, nbc = nexttiled(c->mon->clients); nbc; nbc = nexttiled(nbc->next), n++);
/* Do nothing if layout is floating */
if (c->isfloating || c->mon->lt[c->mon->sellt]->arrange == NULL) {
gapincr = gapoffset = 0;
} else {
/* Apply gaps uniformally. */
gapoffset = gappx;
gapincr = 2 * gappx;
}
/* Adjust the edge gaps. Preferably we'll do this without pushing other windows asside.. */
int edge_x = (x == c->mon->wx) ? edgegap : gapoffset;
int edge_y = (y == c->mon->wy) ? edgegap : gapoffset;
int edge_w = (x + w + 2 * c->bw == c->mon->wx + c->mon->ww) ? edgegap : gapoffset;
int edge_h = (y + h + 2 * c->bw == c->mon->wy + c->mon->wh) ? edgegap : gapoffset;
/* If the window is floating, do not resize it when it reaches the edges. */
if(c->isfloating) {
c->oldx = c->x; c->x = wc.x = x;
c->oldy = c->y; c->y = wc.y = y;
c->oldw = c->w; c->w = wc.width = w;
c->oldh = c->h; c->h = wc.height = h;
} else {
c->oldx = c->x; c->x = wc.x = x + edge_x;
c->oldy = c->y; c->y = wc.y = y + edge_y;
c->oldw = c->w; c->w = wc.width = w - gapincr - (edge_x- gapoffset) - (edge_w - gapoffset);
c->oldh = c->h; c->h = wc.height = h - gapincr - (edge_y - gapoffset) - (edge_h - gapoffset);
}
XConfigureWindow(dpy, c->win, CWX|CWY|CWWidth|CWHeight|CWBorderWidth, &wc);
configure(c);
XSync(dpy, False);
}
Great! But what did all that do?
-
Edge Gap Variable:
- edgegap is set to a larger value than gappx to create larger gaps at the edges of the monitor.
-
Conditional Edge Gap Application:
edge_x
andedge_y
are calculated based on whether the window is at the left or top edge of the monitor (x == c->mon->wx
ory == c->mon->wy
).edge_w
andedge_h
are calculated based on whether the window is at the right or bottom edge of the monitor (x + w + 2 * c->bw >= c->mon->wx + c->mon->ww
ory + h + 2 * c->bw >= c->mon->wy + c->mon->wh
).
-
Position and Size Adjustments:
- The
wc.x
andwc.y
are set tox + edge_x
andy + edge_y
respectively to apply the larger edge gaps where appropriate. - The
wc.width
andwc.height
are set tow - gapincr - (edge_x - gapoffset) - (edge_w - gapoffset)
andh - gapincr - (edge_y - gapoffset) - (edge_h - gapoffset)
respectively to ensure the gaps are applied correctly without overlapping windows (yes! this happened to me. In single client conditions, the gap would even push the window over to my second monitor :P).
- The
Looking pretty!
By customising the resizeclient
function, we've managed to achieve uniform gaps between windows and larger gaps at the edges of the monitor. This provides a more visually appealing layout for our tiling windows manager which is quite satisfying.
I've included some pretty pictures below to demonstrate what this now looks like. You can see examples of how it looked previously by visiting my last post on this subject.
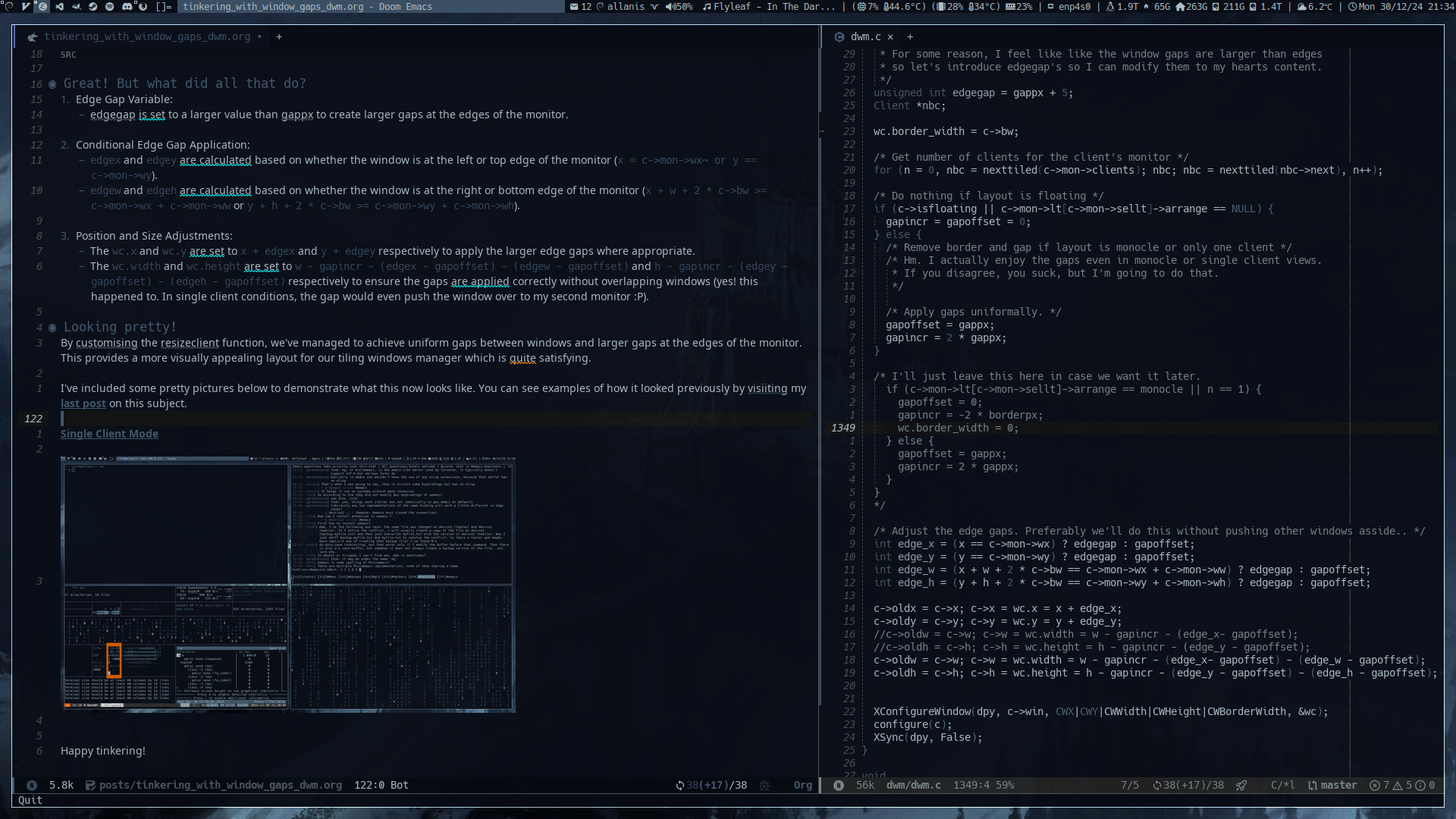
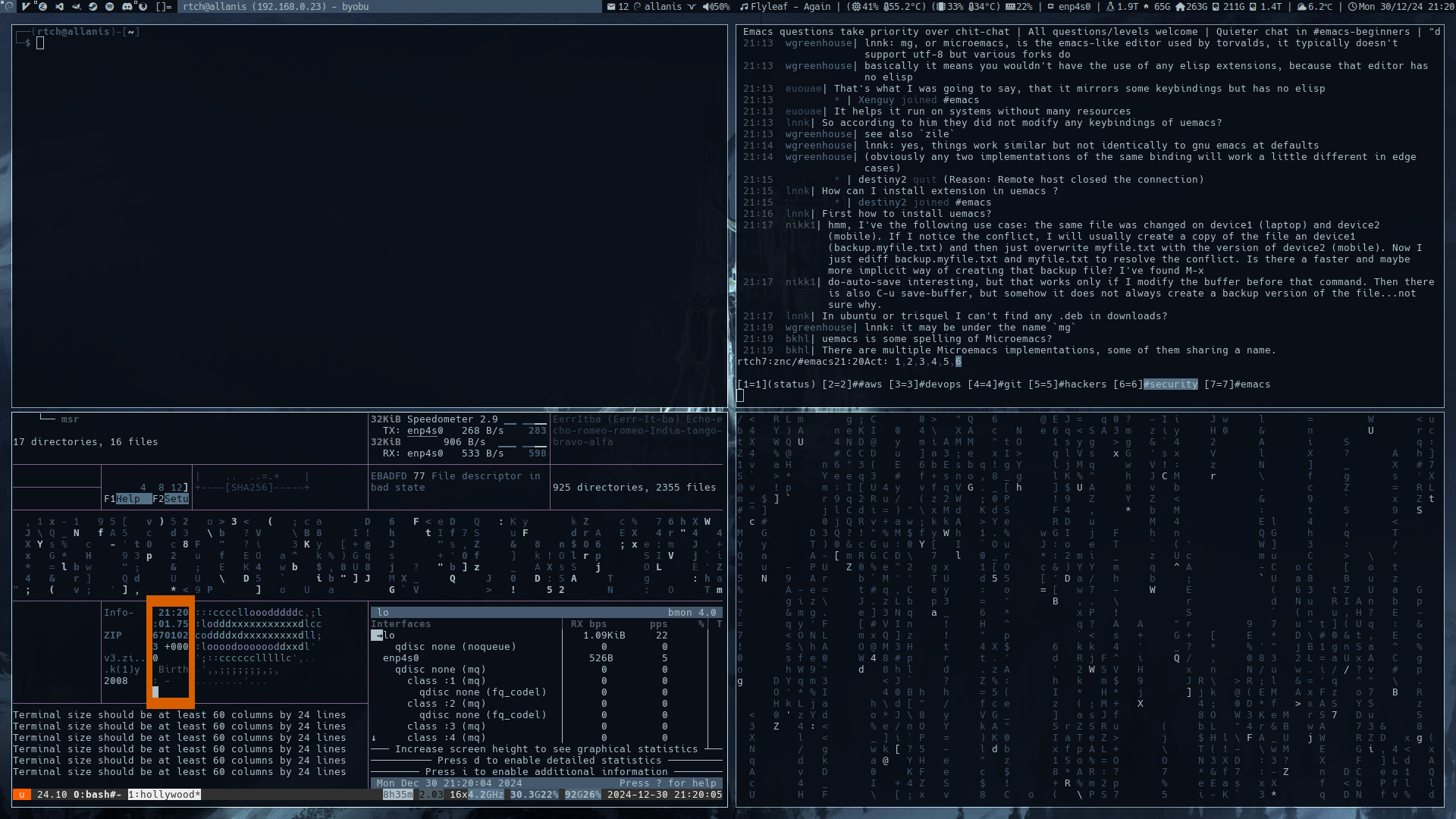
Happy Tinkering.
Addendum
Bugs! Bugs! Bugs!
After implementing the window gaps, I noticed an annoying issue: Windows would shrink when moved to the edges of the screen. This was particularly evident in floating mode and when multiple windows occupied the master stack. In a nutshell, the windows where not respecting their damn boundaries!
To tackle this, I had to ensure that floating windows do not resize when moved to the edges and that gaps are correctly applied for tiled windows, regardless of their arrangement.
How do we fix this?
Well, the solution involved a few changes:
- Floating Windows: I had to ensure that floating windows maintain their size when moved to the edges. We accomplish this by setting the window's position directly without any adjustments for gaps.
if(c->isfloating) {
c->oldx = c->x; c->x = wc.x = x;
c->oldy = c->y; c->y = wc.y = y;
c->oldw = c->w; c->w = wc.width = w;
c->oldh = c->h; c->h = wc.height = h;
/* Handle the top edges. */
if(x == c->mon->wx) wc.x = c->mon->wx;
if(y == c->mon->wy) wc.y = c->mon->wy;
}
- Tiled Windows: I then needed to ensure we are applying edge gaps correctly and that windows do not exceed the edges' boundaries. this involved adjusting the window's width and height to fit within the screen's right and bottom edges (were our problems existed).
if(x == c->mon->wx) wc.x = c->mon->wx + edgegap;
if(y == c->mon->wy) wc.y = c->mon->wy + edgegap;
if(x + w + 2 * c->bw == c->mon->wx + c->mon->ww) wc.width = w - edgegap;
if(y + h + 2 * c->bw == c->mon->wy + c->mon->wh) wc.height = h - edgegap;
/* Respect your damn boundries son! */
if(wc.x + wc.width + 2 * c->bw > c->mon->wx + c->mon->ww - edgegap) {
wc.width = c->mon->wx + c->mon->ww - edgegap - wc.x - 2 * c->bw;
}
if(wc.y + wc.height + 2 * c->bw > c->mon->wy + c->mon->wh - edgegap) {
wc.height = c->mon->wy + c->mon->wh - edgegap - wc.y - 2 * c->bw;
}
Why Handle Top and Left Edges Separately?
The reason for the different treatment of top/left edges versus right/bottom edges comes down to how the resizeclient
function calculates and applies the gaps.
Let's break it down:
- Gap Calculation: We're first determining the gaps for each edge (
edge_x
,edge_y
,edge_w
,edge_h
). we use a larger gap (edgegap
) if the window is flush against the respective screen edge, and a standard gap (gapoffset
) otherwise. -
Applying Gaps: Here's where the discrepancy arises:
- Top and Left: The window's
x
andy
coordinates are simply offset by the calculated edge gaps (edge_x
,edge_y
). This effectively shifts the window inwards, creating the gap. - Right and Bottom: The width and height of the window are reduced to accommodate the gaps. This shrinks the window from all sides. However, if the window is already at the right or bottom edge, shrinking it causes it to overlap the edge, negating the gap.
- Top and Left: The window's
- The Consequence: This difference in how gaps are applied leads to inconsistent behaviour. The top and left gaps work as expected, but the right and bottom gaps effectively disappear when the window is against those edges.
- The Solution: To address this, we include specific checks for windows at the right and bottom edges. We adjust the window's width (
wc.width
) or height (wc.height
) to ensure theedgegap
is correctly applied, preventing overlap and maintaining consistent gaps on all sides.
Now that we've handled these nuances, windows fully respect the edge gaps, and do not shrink when moved to the edges. Now, whether in floating, tiled or monocle mode, the windows behave as expected, maintaining their size and staying within their parameters.
So.. While adding this has been fun and looks (imo) damn sexy! Handling the edge cases (pun intended) probably shouldn't have been overlooked.
Now remember windows:
Respect your damn boundaries son!
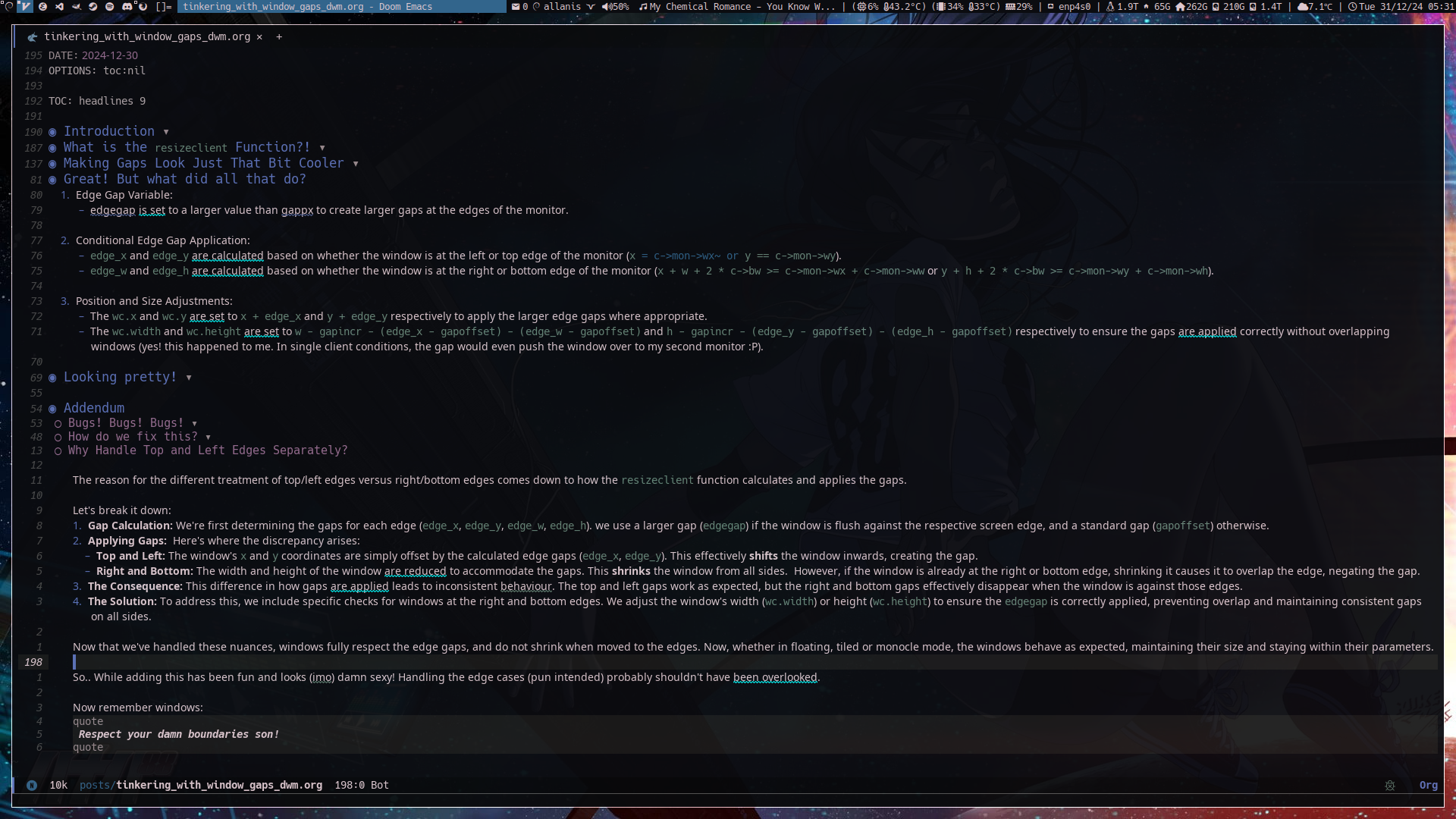